Authentication
Introduction
Subsbase uses JWT as the main means to authenticate any operation (Query or Mutation). The token follows the standard JWT format and it is to be sent with any request in an Authorization
header and preceded by the keywords Bearer
.
Example:
POST https://api.subsbase.io/core/v2/graphql
Authorization: Bearer {your received jwt goes here}
Types of Tokens
There are 2 types of tokens that would be used to interact with the Subsbase Backend:
- Server Tokens
- Customer Tokens (your customer/subscriber)
Server Tokens
Server tokens are specifically intended for backend-to-backend communication purposes. It assumes that the communication is coming from a trusted source and is consumed by your backends.
A server token is requested through:
POST https://api.subsbase.io/auth
X-SITE-ID: {your site id}
The server token could then be used to either Query or Mutate data directly or used to authenticate a customer for further processing.
query GetApiToken {
getApiToken(siteId: "{your site id}", apiSecret: "{your api secret}") {
isSuccess
value
message
}
}
- A
true
value in theisSuccess
fields indicates a successful operation and the token would be in thevalue
field. Otherwise, themessage
would include more information about any error(s) that might have occurred - You can find your API secret in the Settings > Webhook and API Settings page on your Admin Portal.
{your site id}
is the case-sensitive site id you want to use and which contains the plans you need to attach. Your Subsbase Admin Portal link is in the form ofhttps://{your_siteId}.subsbase.io
If you feel the API secret has been comprised during the testing/development phases, you can easily regenerate a new secret in the Settings > Webhook and API Settings page in your Admin Portal.
Customer Tokens
Customer tokens are used to authenticate a specific customer. They are commonly used to enable customers to manage their subscriptions, granting them access to edit, pause, or cancel their plans. The token is expected to be returned to the browser with additional requests originating from the browser within a user's active session.
A customer token is requested through the following Query to https://api.subsbase.io/auth/graphql
.
query GetCustomerToken {
getCustomerToken(
customerId: "{the customerId that will proceed with the token}"
) {
isSuccess
value
message
}
}
- A
true
value in theisSuccess
fields indicates a successful operation and the token would be in thevalue
field. Otherwise, themessage
would include more information about any error(s) that might have occurred.
Data Flow
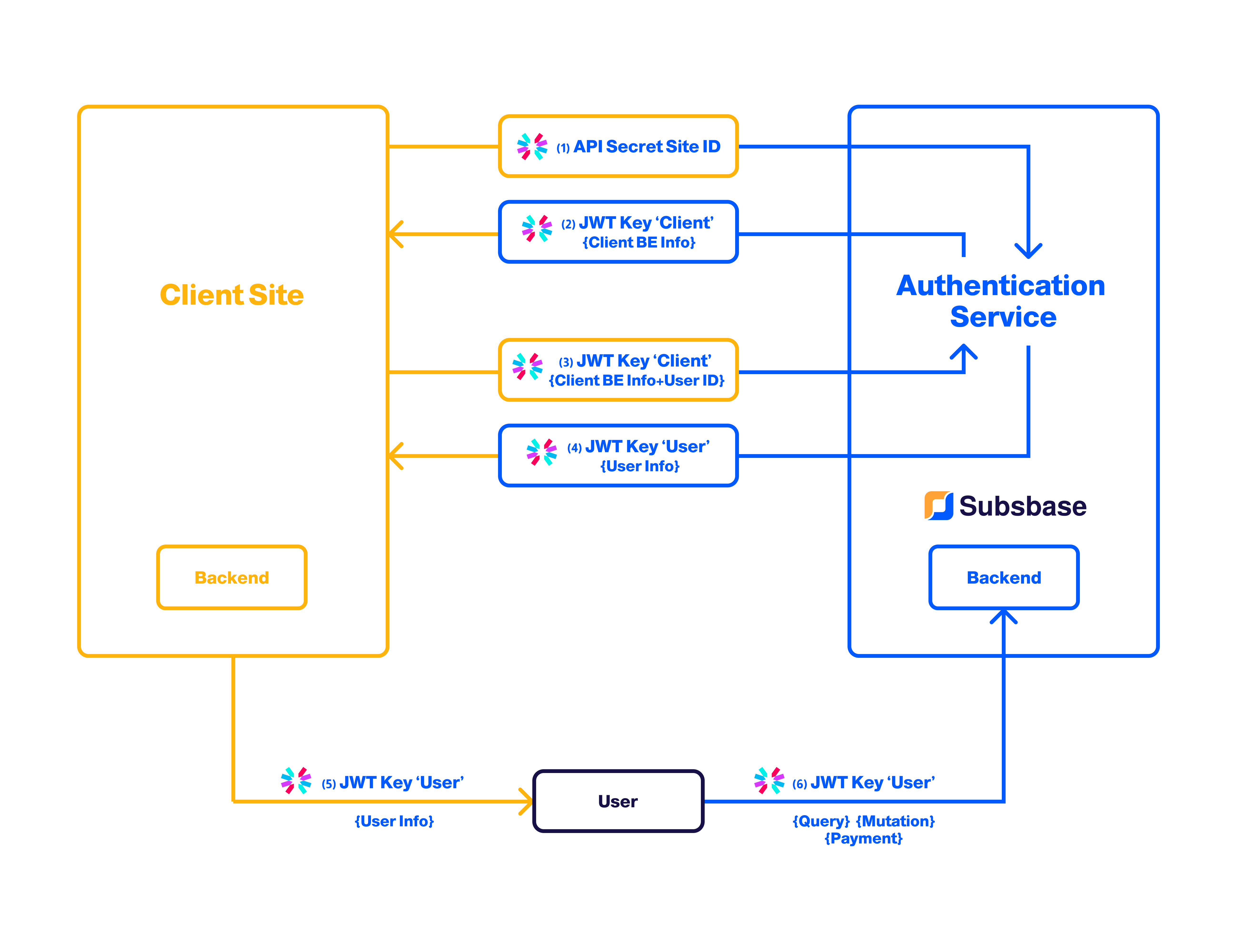